Cross-Site Request Forgery CSRF Attack: 7 Powerful Ways to Prevent It
Cross-Site Request Forgery CSRF is a type of security vulnerability that tricks a victim into submitting a malicious request. This request is initiated without the victim’s knowledge or consent, exploiting the trust that the affected site has in the victim’s browser. Cross-Site Request Forgery CSRF attacks are particularly dangerous because they can compromise user accounts, change settings, or perform actions that the user did not intend to authorize.
Understanding Cross-Site Request Forgery CSRF
How CSRF Works
- Authentication: The victim is authenticated on a target website. This could be through session cookies, tokens, or other authentication mechanisms.
- Malicious Request: The victim visits a malicious website or clicks on a malicious link that triggers an unwanted action on the target site.
- Action Execution: The malicious request is sent to the target site with the victim’s authenticated session, leading to unauthorized actions.
Types of Cross-Site Request Forgery CSRF Attacks
- GET-based Cross-Site Request Forgery CSRF: The malicious request is sent via a GET request, which is often used for retrieving data. For example, changing the email address of a user.
- POST-based Cross-Site Request Forgery CSRF: The malicious request is sent via a POST request, which is often used for submitting data. For example, transferring funds from a bank account.
- Cross-Site Request Forgery CSRF with Multipart Form Data: The malicious request includes multipart form data, which is often used for file uploads.
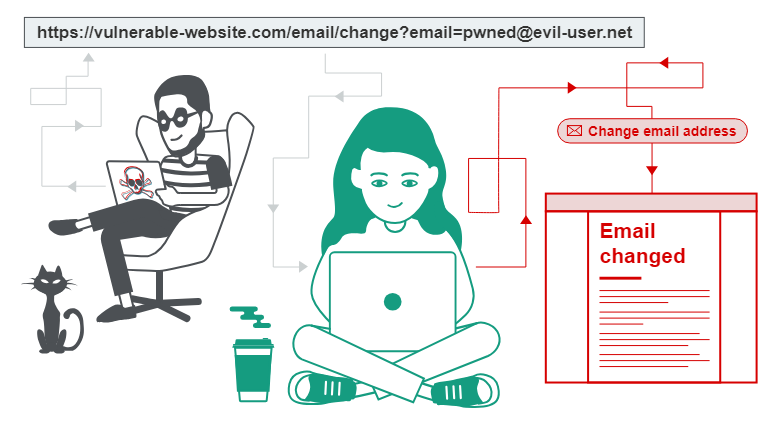
Detecting Cross-Site Request Forgery CSRF Vulnerabilities
Manual Testing
- Identify Endpoints: List all the endpoints that perform state-changing actions (e.g., changing user settings, transferring funds).
- Craft Malicious Requests: Manually craft requests that mimic the actions you want to test. Use tools like Burp Suite or Postman to send these requests.
- Monitor Responses: Check if the actions are executed without proper authorization.
Automated Tools
- Burp Suite: Use the CSRF scanner in Burp Suite to automatically detect CSRF vulnerabilities.
- OWASP ZAP: The OWASP Zed Attack Proxy (ZAP) can also be used to find CSRF vulnerabilities.
- CSRFTester: A specialized tool designed to test for CSRF vulnerabilities.
Mitigating Cross-Site Request Forgery CSRF Vulnerabilities
SameSite Cookies
SameSite cookies are a browser feature that helps mitigate CSRF attacks by controlling how cookies are sent with cross-site requests. There are three possible values for the SameSite attribute:
- Strict: Cookies are only sent in a first-party context and not with requests initiated by third-party websites.
- Lax: Cookies are sent with top-level navigations and will be sent when a user is navigating to the origin site (e.g., by following a link).
- None: Cookies are sent with both first-party and cross-origin requests. This requires the Secure attribute to be set.
httpCopy
Set-Cookie: sessionid=abc123; SameSite=Strict
Anti-CSRF Tokens
Anti-CSRF tokens are unique, secret, and unpredictable values that are generated by the server and included in forms or AJAX requests. The server validates these tokens to ensure that the request is legitimate.
- Generate Token: When a form is rendered, generate a unique token and include it as a hidden field.
- Validate Token: When the form is submitted, validate the token on the server-side.
htmlCopy
<form method="POST" action="/transfer">
<input type="hidden" name="csrf_token" value="unique_token_value">
<!-- other form fields -->
</form>
Custom HTTP Headers
Custom HTTP headers can be used to ensure that requests are coming from a trusted source. For example, the Origin
or Referer
headers can be checked to ensure that the request is coming from the expected domain.
httpCopy
if (request.headers['Origin'] !== 'https://trusted-domain.com') {
return response.status(403).send('Forbidden');
}
Double Submit Cookies
Double Submit Cookies involve sending the CSRF token both as a cookie and as a form field. The server then compares the two values to ensure they match.
- Set Cookie: Set a CSRF token as a cookie.
- Include in Form: Include the same token as a hidden field in the form.
- Validate: Compare the cookie value with the form field value on the server-side.
htmlCopy
<form method="POST" action="/transfer">
<input type="hidden" name="csrf_token" value="unique_token_value">
<!-- other form fields -->
</form>
CAPTCHA
CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) can be used to ensure that the request is coming from a human user. This is not a foolproof method but can add an extra layer of security.
htmlCopy
<form method="POST" action="/transfer">
<input type="text" name="captcha" placeholder="Enter CAPTCHA">
<!-- other form fields -->
</form>
Practical Approach to Mitigating Cross-Site Request Forgery CSRF
Step-by-Step Guide
- Identify Vulnerable Endpoints: Use automated tools or manual testing to identify endpoints that are vulnerable to CSRF attacks.
- Implement Mitigation Techniques: Choose one or more mitigation techniques (e.g., SameSite cookies, anti-CSRF tokens) and implement them.
- Test Mitigation: Test the implemented mitigation techniques to ensure they are effective.
- Monitor and Update: Continuously monitor for new vulnerabilities and update your mitigation strategies as needed.
Example: Implementing Anti-CSRF Tokens
- Generate Token: When rendering a form, generate a unique token and include it as a hidden field.
python
from flask import Flask, request, render_template, session
import secrets
app = Flask(__name__)
app.secret_key = 'supersecretkey'
@app.route('/transfer', methods=['GET', 'POST'])
def transfer():
if request.method == 'POST':
token = request.form.get('csrf_token')
if token != session.get('csrf_token'):
return 'Invalid CSRF token', 403
# Process the transfer
else:
session['csrf_token'] = secrets.token_hex(16)
return render_template('transfer.html', csrf_token=session['csrf_token'])
- Validate Token: On form submission, validate the token on the server-side.
html
<form method="POST" action="/transfer">
<input type="hidden" name="csrf_token" value="{{ csrf_token }}">
<!-- other form fields -->
</form>
Real-World Examples
Example 1: Bank Transfer
A user is authenticated on their bank’s website. A malicious website sends a request to transfer funds to the attacker’s account using the user’s authenticated session.
http
POST /transfer HTTP/1.1
Host: bank.com
Cookie: sessionid=authenticated_session_id
Content-Type: application/x-www-form-urlencoded
amount=1000&to_account=attacker_account
Example 2: Changing Email Address
A user is authenticated on a social media platform. A malicious website sends a request to change the user’s email address.
http
POST /change_email HTTP/1.1
Host: socialmedia.com
Cookie: sessionid=authenticated_session_id
Content-Type: application/x-www-form-urlencoded
new_email=attacker@malicious.com
Conclusion
Cross-Site Request Forgery CSRF is a serious security vulnerability that can compromise user accounts and data. By understanding how CSRF attacks work, detecting vulnerabilities, and implementing effective mitigation techniques, organizations can protect their users and systems from these threats. Regular testing, monitoring, and updating security measures are essential to maintain a robust defense against CSRF attacks.
Additional Resources
- OWASP CSRF Prevention Cheat Sheet: OWASP CSRF Prevention Cheat Sheet
- MDN Web Docs on SameSite Cookies: MDN Web Docs on SameSite Cookies
- Flask Documentation: Flask Documentation
By staying informed and proactive, organizations can effectively mitigate CSRF vulnerabilities and enhance the security of their applications.